Avoid MacOS accent menu
Avoid MacOS accent popup menu
For the Flutter based Terminal application I wanted to deactivate the MacOS behaviour that shows an accent selection popup when a key gets pressed long (instead of repeating the pressed character).
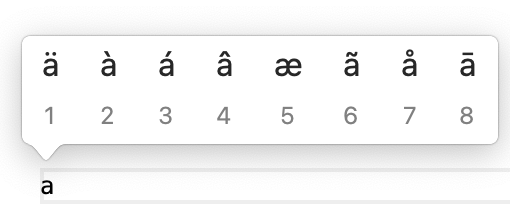
This is actually a feature in MacOS and can be disabled by tweaking some MacOS settings via terminal commands.
I wanted to do that programatically as this behaviour doesn’t make much sense for Terminal applications.
Finding information about this topic is really challanging even if the solution isn’t that complicated so I decided to leave that piece of information here so that someone else might stumble upon it.
Overriding MacOS default settings
The key to the desired behaviour is to override the ApplePressAndHoldEnabled
setting for our application. This can be done by tweaking the integration layer of the Flutter app (the MacOs project).
Just extend the AppDelegate located in AppDelegate.swift
by adding the following method:
override func applicationDidFinishLaunching(_ notification: Notification) {
UserDefaults.standard.set(false, forKey: "ApplePressAndHoldEnabled");
}
That’s all. Now your application doesn’t trigger the accent popup any more.
Read more...